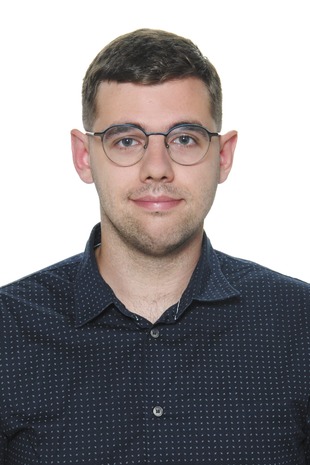
By: Kenan Mičivoda
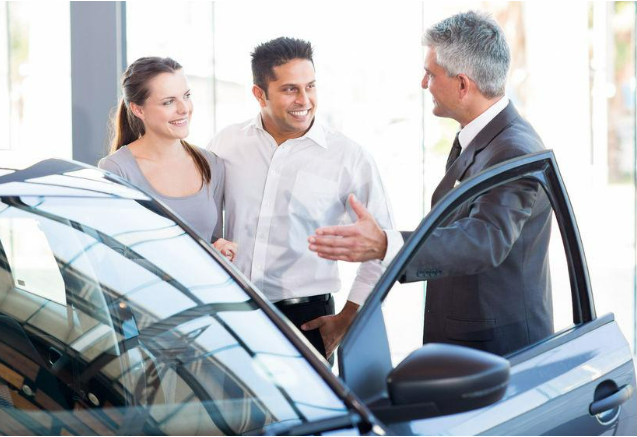
When you consider purchasing a car, you may visualize lengthy conversations with a dealer, reviewing funding alternatives, and arguing on price. What if every step could be completed on its own, between artificial agents, with no human interference? In this section of the blog, I am going to lead you through a Python code that mimics a discussion between a car dealer and a buyer, with the assistance of a financing agent. Using methods from multi-agent systems, this code enables agents to negotiate autonomously, locate common ground, and even determine whether an agreement has been reached. Let’s go over how it works so that everyone, including beginners, may understand the enigma behind it.
What Does the Code Do?
At a high stage, this Python software generates three agents: a dealer, a buyer, and a loan agent who discuss a car sale. The agents speak in a systematic fashion, following predefined dialogue rules and patterns. The negotiation terminates when an agreement is achieved or after a predetermined number of rounds. The code also summarizes their debate and determines whether or not they reached an agreement. Let’s go over each section step by step.
1. Setting Up the Environment
import os
import openai
from autogen import ConversableAgent, GroupChatManager, GroupChat
from dotenv import load_dotenv
load_dotenv()
The first section imports the required libraries, which include OpenAI’s model of languages and “autogen” for multi-agent interactions. The “load_dotenv()” command retrieves environment-specific parameters, such as the OpenAI API key, which is required to access the sophisticated language model. These models stimulate the agents’ “brains,” which allow them to communicate and make conclusions.
2. Agent Class Initialization
class Agents:
def __init__(self):
self.llm_config = {
"config_list": [
{"model": "gpt-3.5-turbo-0125", "api_key": os.environ["OPENAI_API_KEY"]}
]
}
We define a class titled Agents for handling all of our agents. This class includes the agents’ setup, discussions between them, and other beneficial characteristics like summarizing and determining whether an agreement has been achieved. Here, we’re establishing the OpenAI language model, choosing the type of model, and entering the API key for making calls to OpenAI’s services.
3. Agent – specific loading prompts
@staticmethod
def load_prompt(file_path):
with open(file_path, 'r') as file:
return file.read()
This function loads each agent’s “character” from a text file. Each agent receives a cue that tells them how to behave during the negotiation process. For example, the seller may be urged to make profitable offers, but the buyer may be instructed to request discounts.
4. Creating Agents
def create_agent(self, name, prompt):
return ConversableAgent(
name=name,
system_message=prompt,
llm_config=self.llm_config,
max_consecutive_auto_reply=5,
is_termination_msg=lambda msg: "GOOD BYE" in msg["content"],
human_input_mode="TERMINATE",
)
The “create_agent()” function accepts a name and a prompt to create an agent that can take part in discussions. Each agent has a maximum number of ongoing responses it may provide (5 in this case), and the discussion can conclude when the specific closing message is displayed. This makes the system evolving, allowing the agents to interact naturally and stop at the appropriate time.
5. Setting Up a Group Chat
def create_group_chat(self, agents):
return GroupChat(
agents=agents,
messages=[],
max_round=6,
send_introductions=True,
)
This function gathers all agents together in a group chat to exchange messages and negotiate. To prevent the chat from running indefinitely, it is limited to six rounds of discussion. By setting “send_introductions” to “True” value, the agents start by introducing themselves to each other, imitating their first meeting.
6. Summarizing the Conversation
def summarize_conversation(self, chat_result):
conversation_text = "\n".join(
[f"{msg.get('role', 'unknown')}: {msg['content']}" for msg in chat_result.chat_history])
return self.summarize_text(conversation_text)
def summarize_text(self, text):
response = openai.chat.completions.create(
model="gpt-3.5-turbo-0125",
messages=[
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": f"Please summarize the following text:\n{text}"}
],
max_tokens=150,
temperature=0.01
)
return response.choices[0].message.content
After the interaction, we would like to describe what the agents talked. The function “summarize_conversation()” captures each message sent during the discussion and sends them into the OpenAI API to generate a brief overview. This provides a rapid summary of the whole negotiation without having to read through all of the chat records.
7. Checking for Agreement
def check_agreement(self, chat_result):
response = openai.chat.completions.create(
model="gpt-3.5-turbo-0125",
messages=[
{"role": "system", "content": "You are a contract analyst.
Analyze conversation and determine is agreement reached. Return YES or NO."},
{"role": "user", "content": chat_result}
],
max_tokens=100,
temperature=0.01
)
return response.choices[0].message.content
This function validates whether the agents managed to reach an agreement. It sends the chat record to OpenAI’s API, which then examines the dialog to determine whether the negotiation resulted in an agreement to purchase. The answer is either “YES” or “NO”, making it clear whether or not the agents succeeded in negotiating the car sale.
8. The Negotiation in Action
if __name__ == '__main__':
agents = Agents()
seller_prompt = agents.load_prompt('prompts/first_agent_prompt.txt')
customer_prompt = agents.load_prompt('prompts/second_agent_prompt.txt')
finance_prompt = agents.load_prompt('prompts/third_agent_prompt.txt')
seller_agent = agents.create_agent('Seller', seller_prompt)
customer_agent = agents.create_agent('Customer', customer_prompt)
finance_agent = agents.create_agent('Finance', finance_prompt)
group_chat = agents.create_group_chat([seller_agent, customer_agent, finance_agent])
group_chat_manager = GroupChatManager(
groupchat=group_chat,
llm_config=agents.llm_config
)
chat_result = group_chat_manager.initiate_chat(
customer_agent,
message="I am looking for a car, can you provide the best offer?"
)
print("Final Chat Result:", chat_result)
conversation_summary = agents.summarize_conversation(chat_result)
print("Conversation Summary:", conversation_summary)
agreement_status = agents.check_agreement(conversation_summary)
print("Agreement Status:", agreement_status)
The final piece connects everything together. The agents (Seller, Customer, and Finance) load their distinct personalities from text documents and participate in a group discussion. The customer begins the negotiation with a statement “I am looking for a car, can you provide the best offer?” The conversation then proceeds autonomously. Finally, the system prints out the chat summary and if the agents made an agreement.
Example and Explanation of the Possible Output
The customer begins by requesting the greatest deal on an automobile. The seller representative reacts by offering a selection of vehicle models, pricing options, and encouraging the customer to talk about the price. Following a few interactions, the finance agent steps in to offer several financing solutions. For instance, consider first offer a monthly installment of 700 KM for 36 months, with a down payment of 7000 KM and a 5% interest rate. Then, second offer could be monthly installment of 800 KM for 24 months, with a 6000 KM down payment and 4.5% interest rate. And finally, third offer monthly installment of 900 KM for 12 months, with a 5000 KM down payment and 4% interest rate. The customer eventually accepts the second offer, and both sides express satisfaction with the transaction. The output concludes with the summary of their entire conversation and proof that both sides made an agreement.
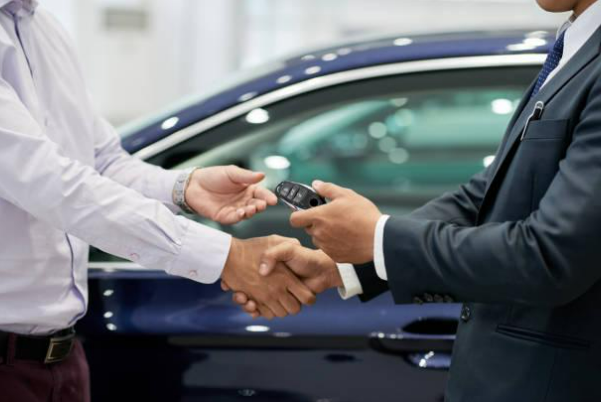
Why Is This Example Innovative?
This code demonstrates how AI can handle difficult conversations autonomously, potentially revolutionizing how organizations function. Instead of extensive back-and-fourths between humans, agents can complete the process easily, fairly, and efficiently. This technology ensures that key tasks can be completed without the need for human involvement by combining AI agents and well-structured language patterns.
The primary innovation here is the utilization of multi-agent decision-making systems. The agents can talk, justify, and discover common ground entirely on their own. Consider developing this program to handle negotiations in industries such as real estate, insurance, and business contracts!
In summary, the possibilities are limitless. Multi-agent systems have a bright future ahead of them, and it is only a matter of time before they are extensively adopted across sectors.
References
- OpenAI. (2023). ChatGPT: Applications and Use Cases. Retrieved from https://openai.com/index/chatgpt/
- py-autogen. (2023). py-autogen Documentation. Retrieved from https://microsoft.github.io/autogen/0.2/docs/Getting-Started